My Ultimate LeetCode Tricks
Check out the LeetCode tricks, formulas, and templates that I used to crack the FAANG interview! Get your hands on the comprehensive collection of my LeetCode cheat sheet, study guide, tricks, and more for FREE!👇
The Free LeetCode Study Guide
Hey Pirates 🏴☠️ PK here. Today, I’m sharing all my LeetCode tricks, the secrets, formulas, and templates that I used to break into FAANG. And guess what? I compiled a complete list of ALL essential techniques on my website - for FREE. No emails, subscriptions, or any bull💩 like that. Just click here. Again, everything you need from the handy LeetCode tricks, a cheat sheet of all the common patterns, their time complexities, the baseline templates, and even LeetCode playground links where you can compile, run, and test the codes yourself in both Python and Java, is all there - for FREE. All you need? Click here, period. Enough talking. Let’s dive into it.
I broke this post down into three sections.
I’ll go over the six tips and tricks that can come in handy during coding interviews.
I’ll go over my LeetCode cheat sheet, and
Show you how to play around with the example codes on my website.
So, let’s start with one, the handy LeetCode tips and tricks.
Tip 1. Leftmost Binary Search
In case you forgot, a binary search is a logarithmic search algorithm that finds the position of a target value within a sorted array. If the problem states that the input array is sorted, there’s a high chance it’s a binary search problem.
Now, this variant of binary search is handier than the conventional one we’ve learned in school because it has two interesting properties besides finding the target.
One, it returns the target’s first occurrence or leftmost index if it exists.
Two, it returns the index of where the target should be if it does not exist.
Let’s go through some examples together. Given this sorted array: [1, 2, 3, 3, 3, 6, 9],
the leftmost binary search of target three returns index two because that’s the first occurrence of the target value. What if the target doesn’t exist, though? If we do a leftmost binary search of target 4, it returns index 5, which is a great location to put the target if we insert it into the sorted array. Now, how is this useful, then? Well, did you notice the leftmost binary search of target three returns its starting index while that of target + 1 returns its ending index + 1? Yes. Within a sorted array, you can use the leftmost binary search to find an element’s first and last position in logarithmic time. Again, you can find this implementation, my solution to the LeetCode problem, a link to the sandbox, and the list of related questions here.
Tip 2. Deque
Instead of memorizing the interfaces of a stack, queue, and linked list, just use a deque! I explain in this video the reasons why I recommend Python and Java for LeetCoding. The two languages shine because Deque is built-in, meaning it might not be available in other languages. A deque is a double-ended queue that can add or remove elements from both ends in amortized constant time.
A Deque can be used as a Stack, Queue, and a Linked List with four easy-to-remember interfaces: addFirst, addLast, removeFirst, removeLast
If you add last and remove last, that’s a stack. If you add last and remove first, that’s a queue. If you do both, that’s a linked list. Do you see how a deque can represent all three data structures with just four interfaces? A word of caution here. Some interviewers, even those at FAANG, do not know what a deque is. 🤦 If you meet one, you might have to explain what it is during the interview or just consider yourself unlucky. 😮💨🤦
Tip 3. String Concatenation
This mistake is one of the most common ones I see. Do not concatenate strings within a loop. Why? String is immutable, meaning you can’t change it once created. When you concatenate two strings, it’s not simply adding one at the end of another. What’s happening internally behind the scenes is that it creates a whole new string; that’s linear, not constant. Solution? Use a StringBuilder instead. It was made specifically for this purpose - to concatenate strings in constant time; it’s virtually a list of characters. For Python users, use string.join() on a list for the equivalent effect.
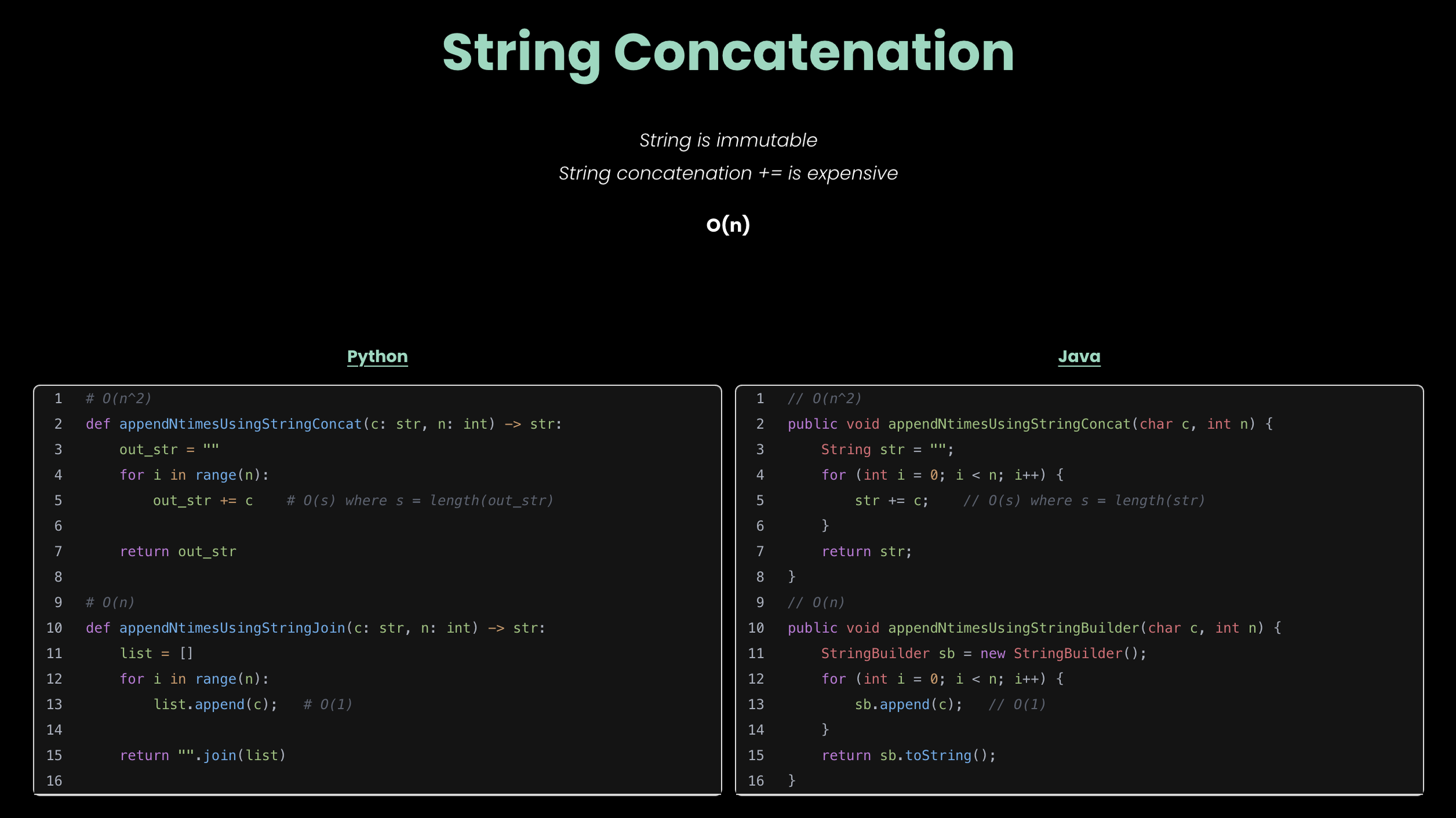
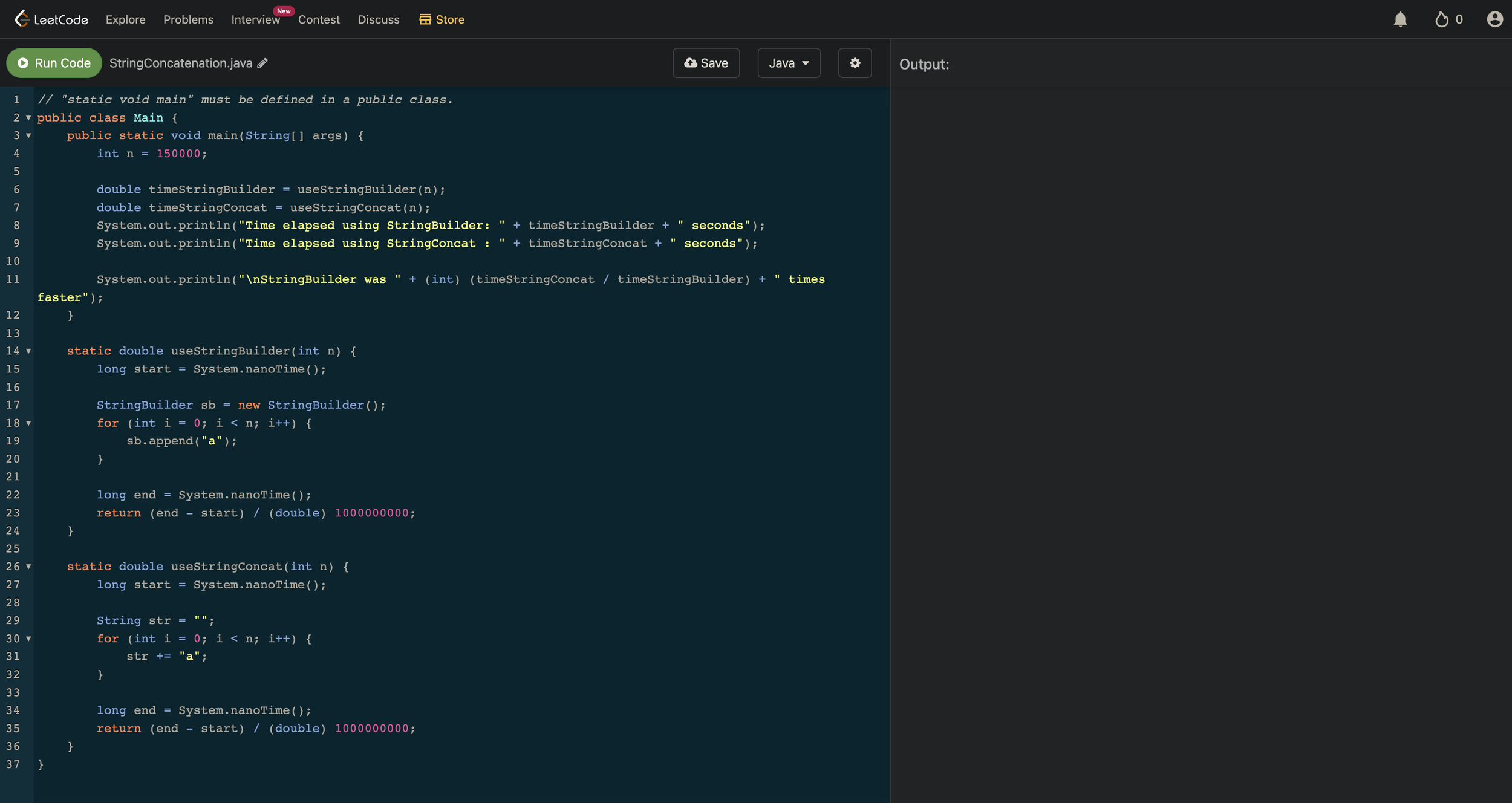
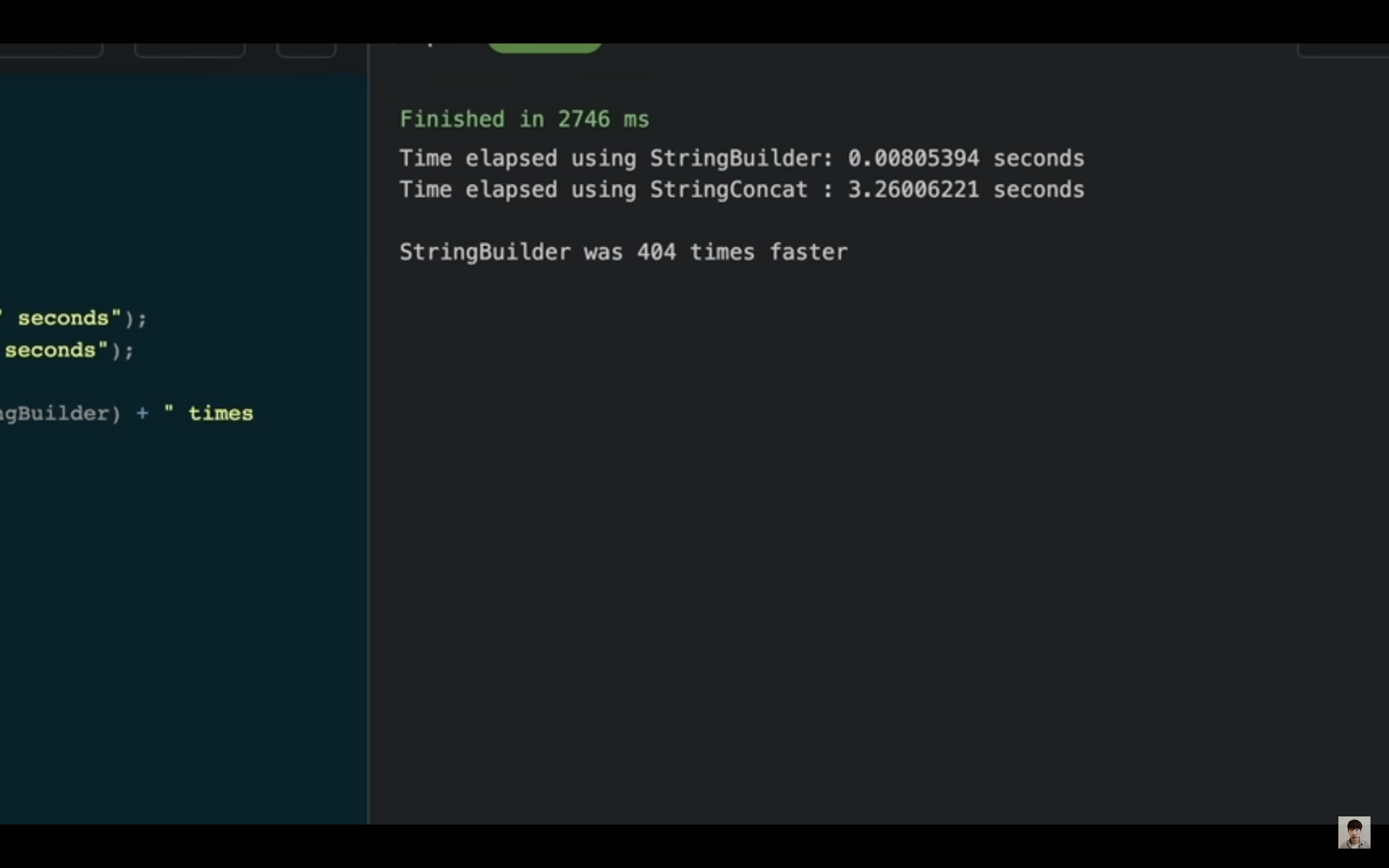
Let me demonstrate how efficient StringBuilder is compared to the += operators. In this LeetCode playground, I’m appending the character ‘a’ n times or 150,000 times as an example. Let’s measure the performance of the same operation for both. Do you see how StringBuilder is significantly faster? Time complexity-wise, the StringBuilder implementation is O(n), while its concatenation counterpart is O(n²). If you’d like a more detailed explanation, google up string immutability.
Tip 4. Inorder Traversal of a Binary Search Tree
Remember binary search tree? It’s a binary tree where the left child is smaller, and the right child is greater than its parent; I’m sure you remember this. But what many people often forget is that the inorder traversal of a binary search tree visits all the nodes in ascending order! You can use this property to validate whether a tree is a binary search tree and retrieve its values in sorted order.
Tip 5. Helper Pointers in a Linked List
If pointer calculations mess up the code, try creating a dummy node; link it to the head. It can simplify your logic and thought process. Also, use slow and fast pointers if the problem requires finding the middle or the nth node (and a cycle) in a Linked List.
Tip 6. Quick Select
Wait, you’ve heard of quick sort, but what’s a quick select? A quick select is a selection algorithm to find the kth smallest or kth largest element in an unordered list. You might say, “Well, that’s easy. I’ll use a PriorityQueue to solve it in O(n log k) time.” True, but can we do better than O(n log k)? This is where quick select comes into play. It works similarly to how quick sort works.
It selects a random pivot and partitions the elements into two groups of less than and greater than the pivot. But unlike quick sort, where you have to process both the left and right subarrays, quick select only needs to do it for one. Think about it. When searching for the largest element, if the pivot ends up in the middle, do we need to involve the smaller half? 🤔 You get the concept, right? Now then. How is this better than O(n log k)? As in the example, if we assume the algorithm recurs half the list each time, it processes n elements in the first recursive call, n/2 in the second, n/4 in the third, and so forth, until there’s only one element remaining. Remember this geometric series?
1 + (1/2) + (1/4) + … + (1/n) = 2
It converges into two. If we multiply it by n,
n + (n/2) + (n/4) + … + 1 = 2n
it converges into 2n or O(n). Yes, for this problem, quick select is the better choice over a priority queue, because the algorithm’s linear on average. The catch here is that it has a worst-case time complexity of O(n²), which happens if the array is already sorted. I won’t discuss the details in this video, but I encourage you to learn why. In real-world implementations, however, quick select is fast and efficient. Understanding how its worst-case performance is quadratic can be a bonus point for your interviews. 😉
LeetCode Cheat Sheet
So these are my six handy tips and tricks. Let’s quickly go over my LeetCode cheat sheet. There are two sections to it: patterns and templates. Patterns is where you’ll find the common problem types, the keywords, the techniques involved, and their time complexities. Click on the links, and you’ll jump to the templates of essential patterns and algorithms. They are in Python and Java, and if you click on the link, it’ll bring you to a playground where you can readily run example codes. If you’d like to modify it, fork the playground. Easy, right?
Cool. Just an FYI, I created this page with the initiative to help you guys get started on LeetCode. I hate to say this, but I’ll be fully transparent and say that this alone won’t be enough. LeetCode is hard, which is why even engineers at FAANG grind months in advance; it takes time and effort. This cheat sheet is just a baseline and not a shortcut to acing an interview. Recall the cheat sheet you created during your college exam. You must prepare for the test to make full use of it, right? On the other hand, going to an exam without studying but with someone else’s cheat sheet is like walking into your own grave. Know your algorithms. Grind, grind, and grind. Most importantly, don’t give up. Months of hard work will pay off.
Summary
That’s it, pirates. I tried to pack my cheat sheet with as many comprehensive details as possible. Let me know in the comments below if I’m missing anything or if you have any ideas or suggestions. I also compiled a list of relevant questions, study guide, and excellent resources to accelerate your grind, so check it out. Lastly, while surfing my homepage, you might as well explore some great deals, including $1,000 off any springboard bootcamps and free stocks on webull. Make sure to like and subscribe. Thanks for watching. I’ll see you at the next one. Peace 👑